Building Applications with Azure Functions
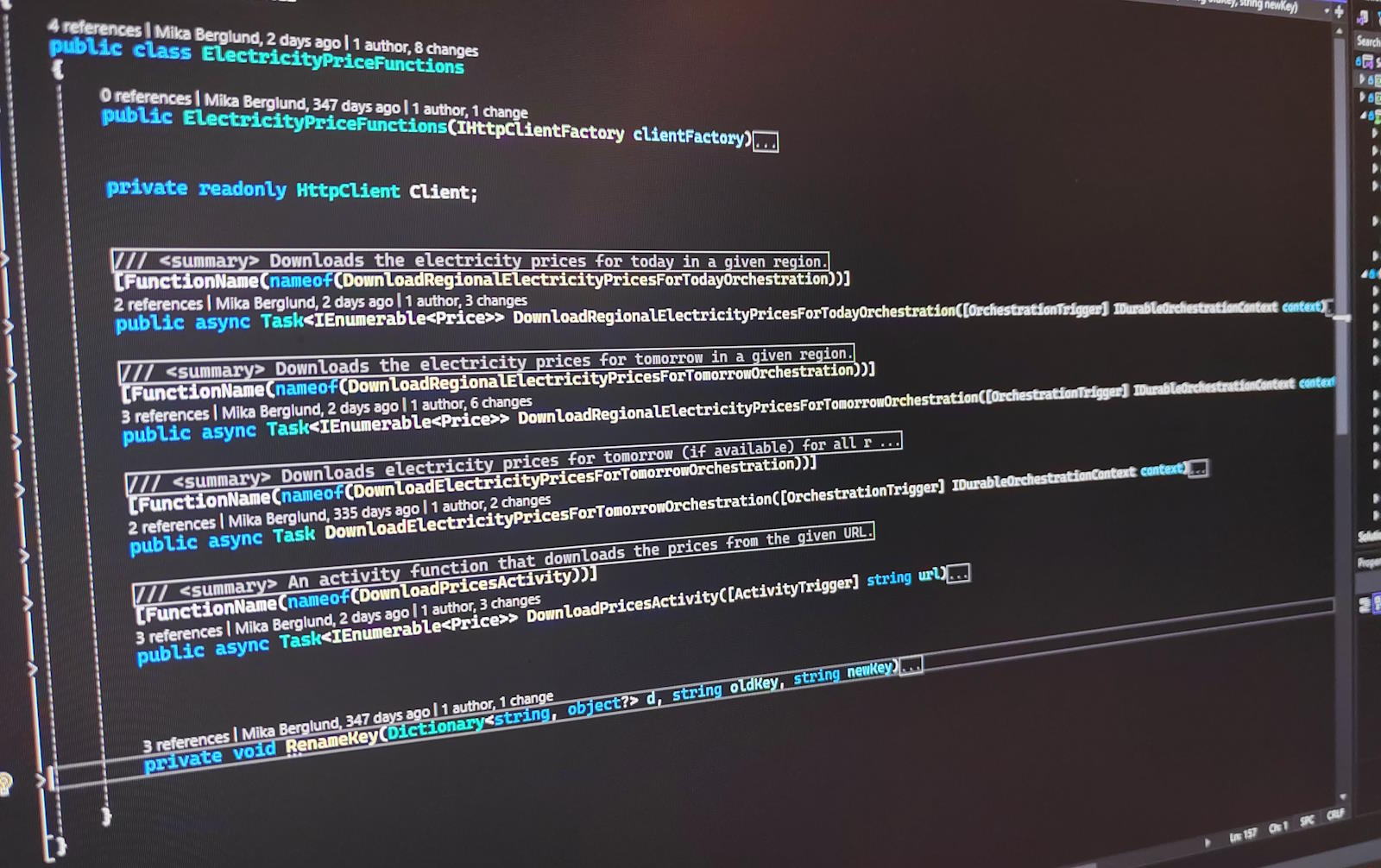
Azure Functions is probably one of the first serverless service in Azure. It might even be the first. The idea in Azure Functions is pretty simple. You just write one or more functions, that you then trigger in various ways. You can trigger your functions in response to various external events. For instance a blob landing in blob storage, a message arriving in a Service Bus queue, or in response to HTTP requests.
In this post I talk about the things I think are good to know before setting out to build applications with Azure Functions.
I also created a GitHub repository with sample code to go along with this post. Make sure you check that out too.
Creating a Function Application
There are a lot of good documentation on how to create Azure Functions applications, like this one. That’s why I’m not going to go into details about creating a function application.
There are a few things I’d like to point out though. At the time of writing, mid-September 2019 using Visual Studio 2019 (v16.2.5) the Azure Functions template still creates one static class with one static function method for each function you create.
In my Azure Functions with Dependency Injection tutorial on GitHub, I wrote that instance methods have been supported in Azure Functions for a while now. Instance methods implies that you need to create instances of your classes, meaning that your classes have constructors. And constructors build the foundation for Dependency Injection in both ASP.NET Core and Azure Functions.
With Dependency Injection you can for instance simplify your Function Application configuration, as I’ve shown you in another tutorial I wrote on GitHub, Azure Functions Configuration.
Naming Functions
When writing functions for Azure Functions, each function method has to be decorated with the FunctionName
attribute. This attribute tells the Functions runtime that the method is a function and defines the name for the function. Each name must be unique across your entire application.
To avoid using string constants as function names with the FunctionName
attribute, I’ve adopted a habit where I use the name of the actual function method as the name for the FunctionName
attribute using the nameof
operator, as shown in the code sample below.
[FunctionName(nameof(GenericWebhookCallbackHttp))] public async Task<HttpResponseMessage> GenericWebhookCallbackHttp( [HttpTrigger("GET")] HttpRequestMessage request ) { ... return new HttpResponseMessage(...); }
This becomes particularly useful when you start referencing other functions in code, for instance when writing durable functions, which I’ve written another post about. But even with just standard functions, you get the benefit of ensuring that your function names are unique, and stay unique. And you don’t have to resort to magic strings in your code.
Configuration
With Dependency Injection, configuration is very easy to manage. The key thing here is to model your configuration data as one or more classes that your configuration settings then reflect. In your startup class, you then convert the configuration settings into class instances, and inject them as services too.
To get you going in the right direction, have a look at my Azure Functions Configuration tutorial on GitHub.
Summary
It’s really easy to fire up a new Azure Functions application and start coding, but I feel that the templates in Visual Studio still leave a bit to desire, which is why I wrote this post.
To wrap things up, here are a few bullets I hope you remember when creating Azure Functions applications.
- Create your function methods as instance methods in non-static classes.
- Create shared functionality as services that you inject in your startup class.
- Group several functions into classes based on their dependency, and have the dependencies injected in the class constructors.
- Create one or more classes that represent your configuration and inject them as services too.
- Use constants as names for your functions instead of magic strings.
Have fun with Azure Functions! I know I have!
0 Comments