JavaScript Interop Error in Blazor – JSException: Unexpected token ‘?’
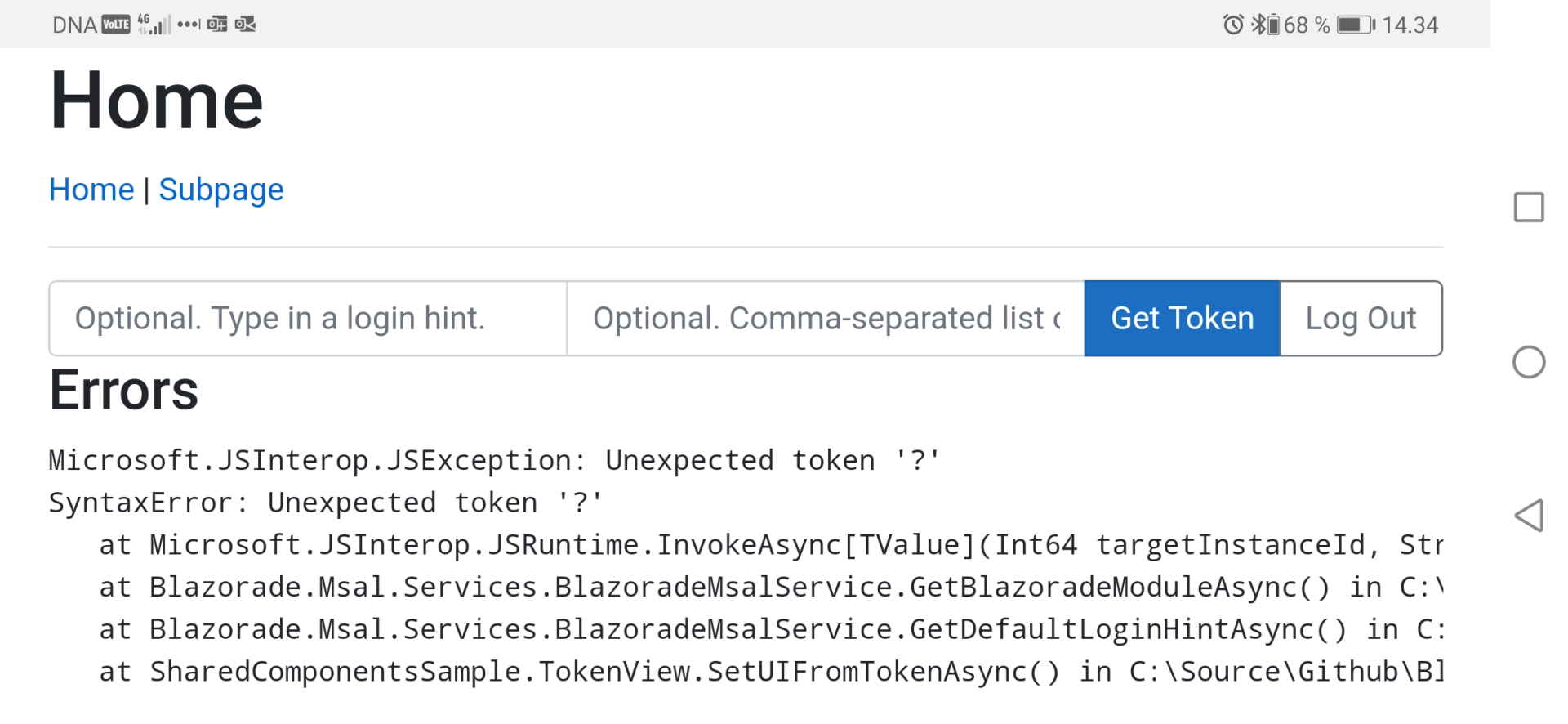
I am working on getting the first stable version of Blazorade MSAL ready and published. While I was testing the code on various browsers and devices, I ran into a problem when running on Microsoft Edge for Android, which did not affect any other platforms I tried.
In this article I’ll describe the problem and solution in detail, so that you don’t have to bang your head against the wall trying to figure out the solution.
The Problem Description
As I wrote above, the problem occurred only when running in Microsoft Edge for Android. I did not try on other mobile devices. In Chrome on the same device, everything worked just fine. Also, on Windows desktop both Edge and Chrome worked just fine too.
I pinpointed the problem to the code where I was importing a JavaScript file for interop calls. The problematic code line is highlighted below.
private IJSObjectReference _BlazoradeModule; private async Task<IJSObjectReference> GetBlazoradeModuleAsync() { var msalModule = await this.GetMsalModuleAsync(); return _BlazoradeModule ??= await this.JSRuntime.InvokeAsync<IJSObjectReference>( "import", "./_content/Blazorade.Msal/js/blazoradeMsal.js" ).AsTask(); }
The error that I got was:
Microsoft.JSInterop.JSException: Unexpected token '?' SyntaxError: Unexpected token '?' at Microsoft.JSInterop.JSRuntime.InvokeAsync[TValue](Int64 targetInstanceId, String identifier, Object[] args) at Blazorade.Msal.Services.BlazoradeMsalService.GetBlazoradeModuleAsync() in \Blazorade.Msal\Services\BlazoradeMsalService.cs:line 330 at Blazorade.Msal.Services.BlazoradeMsalService.GetDefaultLoginHintAsync() in \Blazorade.Msal\Services\BlazoradeMsalService.cs:line 160 at SharedComponentsSample.TokenView.SetUIFromTokenAsync() in \SharedComponentsSample\TokenView.razor:line 78
The obvious initial thought was that Edge did not understand the null-coalescing assignment operator ??=
. However, after some additional thoughts, I did not suspect that for long, since that is the .NET code that is running in the same .NET runtime. Of course I tried to write the code without the ??=
operator, but as expected, it was not the cause.
A Simple Solution
After various attempts to rewrite my code and try it on Edge mobile, I finally found the cause. In my JavaScript code I was using the null-coalescing operator ??
, as shown below.
msalConfig.auth = msalConfig.auth ?? {};
Seems that this works on all browsers I tested it on, except for Microsoft Edge on Android. As I already wrote above, the same problem did not affect Chrome on Android. Just Edge. Chrome and Edge on Windows worked just fine.
The fix was quite easy at the end of the day. I just changed the code above to using inline IFs, as shown below.
msalConfig.auth = msalConfig.auth ? msalConfig.auth : {};
That fixed the problem, and now it works consistently on all browsers that I’m testing it on. Hope this can help you if you run into a similar problem, and can’t figure out what the cause is.
0 Comments