Customizing Connection State UI in Blazor Server Applications with Blazorade
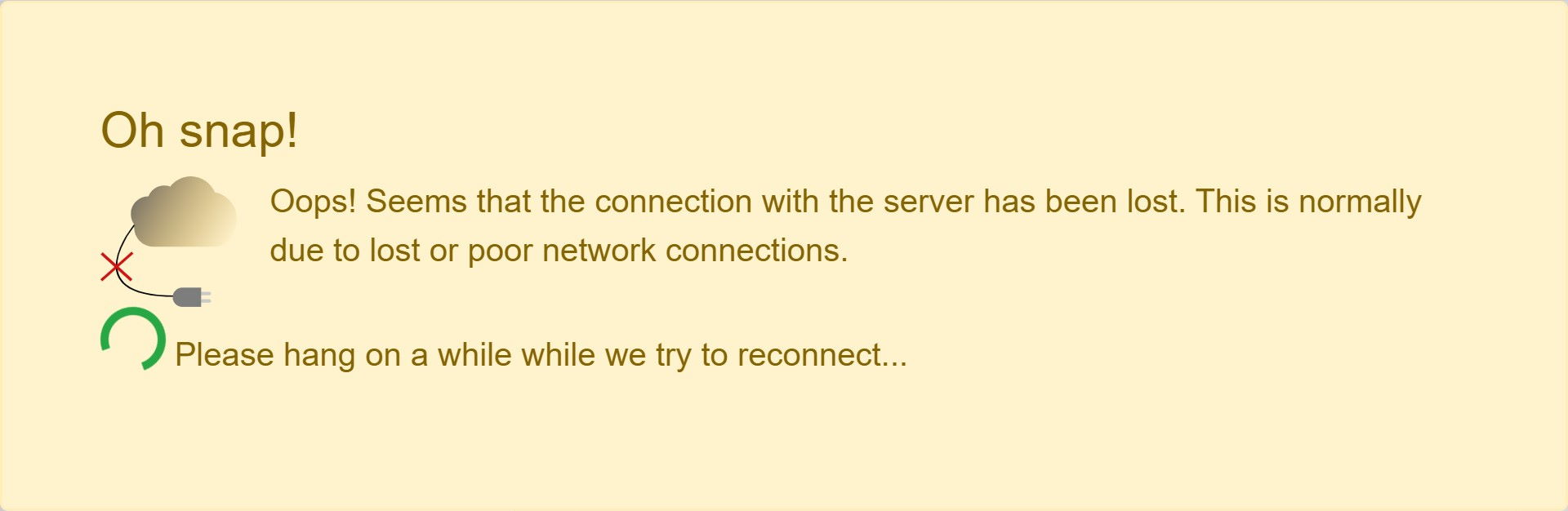
If you’ve been working with Blazor Server applications, you might have come across the message shown below.
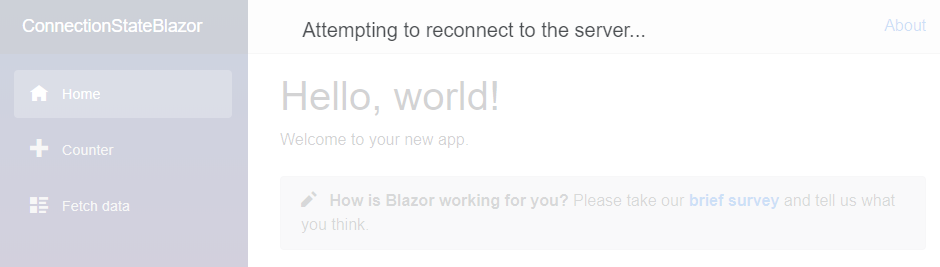
A Blazor Server application shows this message whe it looses it’s connection with the server. Remember that Blazor Server uses socket connections handled by SignalR to communicate with the server. If these connections are broken, the application cannot work properly, so it shows the message above.
There are a few other alternatives to the message, which an application shows in different situations, like the one below.
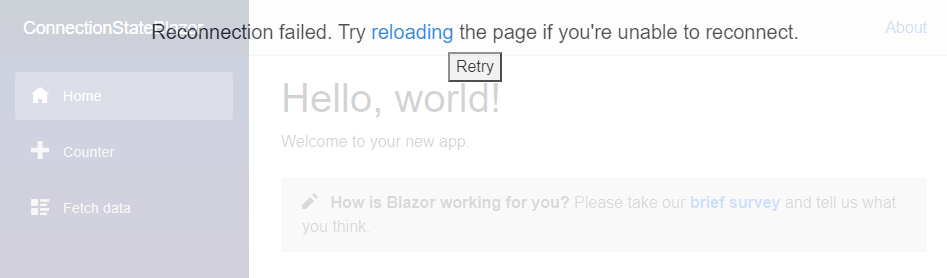
Regardless of the alternatives, it is quite clear that you might want to customize these messages. Luckily there is a way, which is described here. However, it might be a bit tricky, so I decided to create a component for it, and include it in Blazorade.Core as the ComponentReconnectModal
component.
This article is about how to use that component in your own Blazor Server application.
Getting Started
The first thing you need to do is to add the reference to the package. It is available on Nuget, so the simplest way is to just run the following command in your package manager console.
Install-Package -Id Blazorade.Core
You can naturally also install it using Visual Studio’s UI. When you have the reference, add the following using
statement to your _Imports.razor
file. This is so that you don’t have to reference the component using its full namespace.
@using Blazorade.Core.Components.Server
You can of course place the component wherever you like in your application. Keep in mind though that the component, or a customized version of the component, must always be rendered. The component shows a UI only when there is a problem with the connection. In normal operations the component will not show a UI. You never know what UI your users have rendered when connection problems hit. So keep the component rendered at all times.
I tend to put the component at the end of the App.razor
file, which is the root UI component of your Blazor server application. So, your App.razor
file would then look like this.
<Router AppAssembly="@typeof(Program).Assembly"> <!-- Omitted markup --> </Router> <ComponentReconnectModal> </ComponentReconnectModal>
Customizing the UI
The sample code above would render the default UI provided by the ComponentReconnectModal
component. That’s probably not what you want, since you are reading an article about customizing the UI. The way you customize the ComponentReconnectModal
component is by specifying templates that provide the UI you want to show for the various states, like the code sample below shows.
<ComponentReconnectModal> <ReconnectingTemplate> <p> Oops! Seems that the connection with the server has been lost. This is normally due to lost or poor network connections. </p> <p> Please hang on a while while we try to reconnect... </p> </ReconnectingTemplate> <ReconnectFailedTemplate> <p> We're sorry, but the reconnection attempts failed. </p> <p> You can now either try to <a href="javascript:window.Blazor.reconnect()">reconnect</a> or just <a href="javascript:location.reload()">reload</a> the page. </p> <p> Be prepared that reloading will not work, since failure to reconnect usually is due to a longer network failure or the server has been taken down for maintenance. </p> </ReconnectFailedTemplate> <ReconnectRejectedTemplate> <p> The connection attempts have all been rejected by the server. This is often caused by a fault on the server. </p> <p> You can try to <a href="javascript:location.reload()">reload</a> the page, but please note that this will likely not succeed due to the likely failure on the server. </p> </ReconnectRejectedTemplate> </ComponentReconnectModal>
The templates that are available in the ComponentReconnectModal
component are:
ReconnectingTemplate
– Contains the UI that you want to show when your client initially looses the connection. The client application is attempting to reconnect with the server at this point.ReconnectFailedTemplate
– All attempts to reconnect with the server have failed. Your client application will not automatically attempt to reconnect with the server. At this point, you can either try to reconnect, or simply reload the page and hope that your client application will be able to connect with the server.ReconnectRejectedTemplate
– You client application was able to connect with the server, but for some reason, the server refused the connection. Your only option is to reload the page.
With the help of Blazorade Bootstrap for instance, you could customize your UI to look something like this.
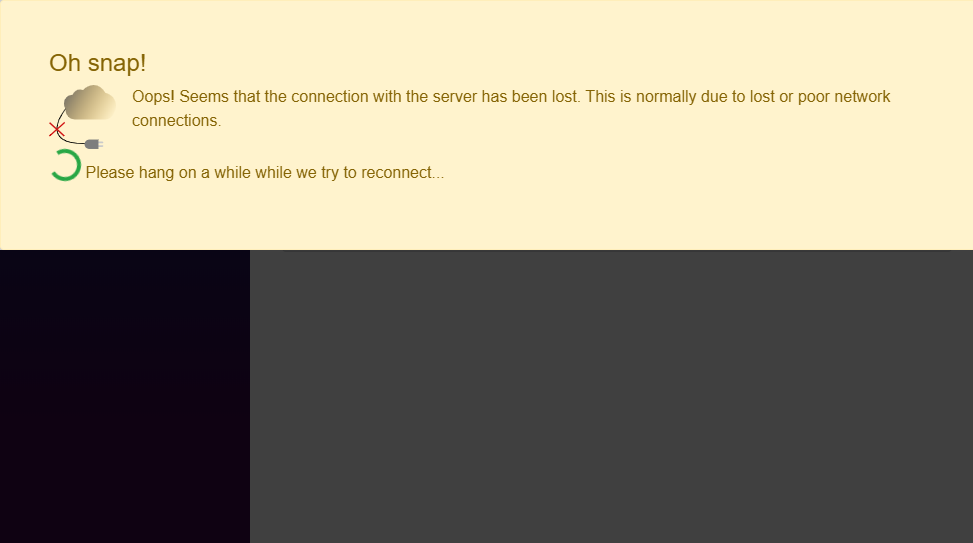
Additional Parameters
The ComponentReconnectModal
component does not only expose the template parameters described above. It also exposes the following parameters that enable easier customization.
BackdropBackgroundColor
– The colour for the backdrop. The backdrop covers your entire application.ConnectionStateBackgroundColor
– The background colour of the area showing the different connection state UIs.ConnectionStatePadding
– The padding for the connection state UI area.
For details on how to use these parameters, please head to the Wiki page for the ComponentReconnectModal
component.
Hope you find this component useful. Also have a look at the Blazorade Bootstrap library, in case you are planning on writing Blazor applications with Bootstrap.
0 Comments